You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
Tell us what label refers to the channels, or colors, in the image.
144
+
### Text and image usage examples
145
+
Lets illustrate the template above with *textual* data. The data item of interest is a sentence being (a part of) a movie review and the model has been trained to classify reviews into positive and negative sentiment classes.
146
+
We are intersted which words are contributing positively (red) and which - negatively (blue) towards the model's desicion to classify the review as positive and we would like to use the *LIME* explainer:
154
147
155
148
```python
156
-
axis_labels = {0: 'channels'}
149
+
model_path ='your_text_model.onnx'
150
+
# also define a model runner here (details in dedicated notebook)
151
+
review ='The movie started great but the ending is boring and unoriginal.'
Run using the XAI method of your choice, for example RISE:
160
+
Here is another illustration on how to use dianna to explain which parts of a bee *image* contributied positively (red) or negativey (blue) towards a classifying the image as a *'bee'* using *RISE*.
161
+
The Imagenet model has been trained to distinguish between 1000 classes (specified in ```labels```).
162
+
For images, which are data of higher dimention compared to text, there are also some specifics to consider:
For visualization of the heatmap please refer to the [tutorial](https://github.com/dianna-ai/dianna/blob/main/tutorials/explainers/LIME/lime_timeseries_coffee.ipynb)
201
-
202
-
### Tabular example:
203
-
204
-
```python
205
-
model_path ='your_model.onnx'# model trained on tabular data
Run using the XAI method of your choice. Note that you need to specify the mode, either regression or classification. This case, for instance a regression task using KernelSHAP with the following additional arguments:
plot_tabular(explanation, X_test.columns, num_features=10) # display 10 most salient features
216
-
```
185
+
### Overview tutorial
186
+
There are **full working examples** on how to use the supported explainers and how to use dianna for **all supported data modalities** in our [overview tutorial](./tutorials/overview.ipynb).
[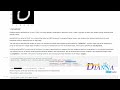](https://youtu.be/u9_c5DJewLU)
219
190
220
191
### IMPORTANT: Sensitivity to hyperparameters
221
-
The XAI methods (explainers) are sensitive to the choice of their hyperparameters! In this [work](https://staff.fnwi.uva.nl/a.s.z.belloum/MSctheses/MScthesis_Willem_van_der_Spec.pdf), this sensitivity to hyperparameters is researched and useful conclusions are drawn.
192
+
The explainers are sensitive to the choice of their hyperparameters! In this [work](https://staff.fnwi.uva.nl/a.s.z.belloum/MSctheses/MScthesis_Willem_van_der_Spec.pdf), this sensitivity to hyperparameters is researched and useful conclusions are drawn.
222
193
The default hyperparameters used in DIANNA for each explainer as well as the values for our tutorial examples are given in the Tutorials [README](./tutorials/README.md#important-hyperparameters).
223
194
224
195
## Dashboard
@@ -252,7 +223,7 @@ DIANNA comes with simple datasets. Their main goal is to provide intuitive insig
| Coffee dataset <imgwidth="25"alt="Coffe Logo"src="https://github.com/dianna-ai/dianna/assets/3244249/9ab50a0f-5da3-41d2-80e9-70d2c8769162"> | Food spectographs time series dataset for a two class problem to distinguish between Robusta and Arabica coffee beans. | <imgwidth="500"alt="example image"src="https://github.com/dianna-ai/dianna/assets/3244249/763002c5-40ad-48cc-9de0-ea43d7fa8a75)"> |[data source](https://github.com/QIBChemometrics/Benchtop-NMR-Coffee-Survey)|
226
+
|[Coffee dataset](https://www.timeseriesclassification.com/description.php?Dataset=Coffee) <imgwidth="25"alt="Coffe Logo"src="https://github.com/dianna-ai/dianna/assets/3244249/9ab50a0f-5da3-41d2-80e9-70d2c8769162"> | Food spectographs time series dataset for a two class problem to distinguish between Robusta and Arabica coffee beans. | <imgwidth="500"alt="example image"src="https://github.com/dianna-ai/dianna/assets/3244249/763002c5-40ad-48cc-9de0-ea43d7fa8a75)"> |[data source](https://github.com/QIBChemometrics/Benchtop-NMR-Coffee-Survey)|
256
227
|[Weather dataset](https://zenodo.org/record/7525955) <imgwidth="25"alt="Weather Logo"src="https://github.com/dianna-ai/dianna/assets/3244249/3ff3d639-ed2f-4a38-b7ac-957c984bce9f"> | The light version of the weather prediciton dataset, which contains daily observations (89 features) for 11 European locations through the years 2000 to 2010. | <imgwidth="500"alt="example image"src="https://github.com/dianna-ai/dianna/assets/3244249/b0a505ac-8a6c-4e1c-b6ad-35e31e52f46d)"> |[data source](https://github.com/florian-huber/weather_prediction_dataset)|
|*Text*|[Stanford sentiment treebank](https://nlp.stanford.edu/sentiment/index.html)|Positive or negative movie reviews sentiment *classificaiton*| <imgwidth="25"alt="nlp-logo_half_size"src="https://user-images.githubusercontent.com/3244249/152540890-c8e1e37d-f0cc-4f84-80a4-2c59176cbf4c.png">|
27
-
|*Timeseries*| Coffee dataset | Binary *classificaiton* of Robusta and Aribica coffee beans| <imgwidth="25"alt="Coffe Logo"src="https://github.com/dianna-ai/dianna/assets/3244249/9ab50a0f-5da3-41d2-80e9-70d2c8769162">|
27
+
|*Timeseries*|[Coffee dataset](https://www.timeseriesclassification.com/description.php?Dataset=Coffee)| Binary *classificaiton* of Robusta and Aribica coffee beans| <imgwidth="25"alt="Coffe Logo"src="https://github.com/dianna-ai/dianna/assets/3244249/9ab50a0f-5da3-41d2-80e9-70d2c8769162">|
28
28
||[Weather dataset](https://zenodo.org/record/7525955)|Binary *classification* (summer/winter) of temperature time-series |<imgwidth="25"alt="Weather Logo"src="https://github.com/dianna-ai/dianna/assets/3244249/3ff3d639-ed2f-4a38-b7ac-957c984bce9f">|
0 commit comments