You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
Update to the latest version of docking and now all the text looks messed up. (See screenshot). Also trying to move the window outside of the main window renders it in purple, it also sometimes crashes my nvidia driver.
This seems to only be a issue with OpenGL since I have tested it on win32 dx9 10 and 11
Screenshots/Video
Standalone, minimal, complete and verifiable example: (see #2261)
Apologies, I broke the GL renderer with that last commit yesterday. It worked when I first tested it then I tweaked variables and the * sizeof() multiplier got removed in the process. Pushed the fix now.
Version/Branch of Dear ImGui
Version: 1.71 WIP
Branch: docking/8dc04a4
Back-end/Renderer/Compiler/OS
Back-ends: imgui_impl_opengl3.cpp + imgui_impl_win32.cpp
Compiler: MSVC 19.20.27508.1
Operating System: Windows 10
My Issue/Question:
Update to the latest version of docking and now all the text looks messed up. (See screenshot). Also trying to move the window outside of the main window renders it in purple, it also sometimes crashes my nvidia driver.
This seems to only be a issue with OpenGL since I have tested it on win32 dx9 10 and 11
Screenshots/Video
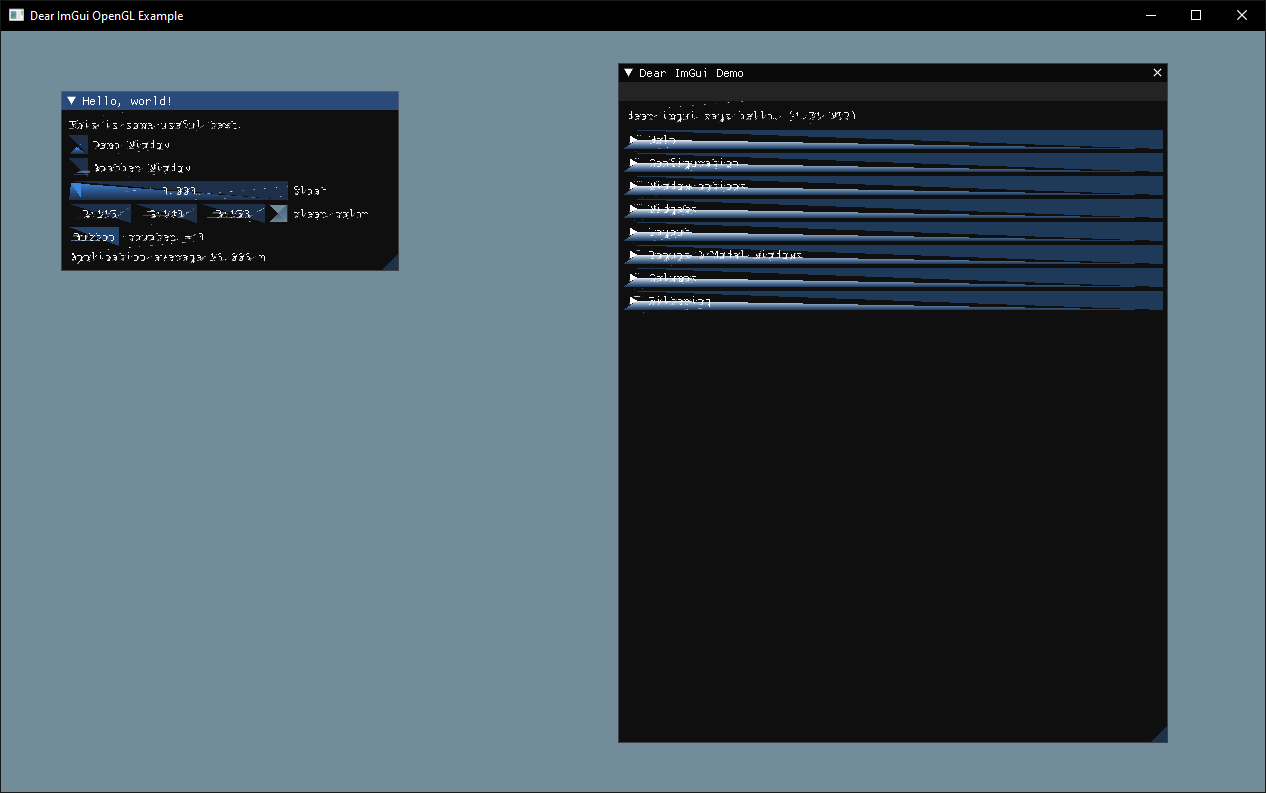
Standalone, minimal, complete and verifiable example: (see #2261)
**Debug output: **
GL_VERSION = "4.6.0 NVIDIA 430.86"
Related to
#2593
The text was updated successfully, but these errors were encountered: